I have had a chance to solve Codility demo test. It is available for free, and you can try it unlimited times. While I do not believe that such tests correlate to typical real-world programming tasks, they are often used as a first interview filter by some companies. Here I show a few Python variations for the solution.
It is fascinating that each programmer solves the same task differently. First two solutions are mine, third belongs to my girlfriend, and last I found on nkbits blog.
Task description
Write a function:
def solution(A)
that, given an array A of N integers, returns the smallest positive integer (greater than 0) that does not occur in A.
For example, given A = [1, 3, 6, 4, 1, 2], the function should return 5.
Given A = [1, 2, 3], the function should return 4.Given A = [−1, −3], the function should return 1.
Dec 01, 2020 Alternative solution for Task 3: def isTriangle (arr): # If the number of elements # is less than 3, then # a triangle isn’t possible N = len(arr) if N arri + 2: return True. When complete, first realize that 'MOON' requires TWO 'Os', so divide the total number of 'Os' found by 2. Then, compare the totals for each of the three letters, seeing which one was the smallest. That should be the 'limiting factor' in how many times I can spell the word. The tests on Codility vary in terms of difficulty. I suspect the employer has a say on which questions you're presented with on your online exam. You can practice with the 'lessons' that they have on their website.
Assume that:
N is an integer within the range [1.100,000];each element of array A is an integer within the range [−1,000,000.1,000,000].Complexity:
- expected worst-case time complexity is O(N);
- expected worst-case space complexity is O(N) (not counting the storage required for input arguments).
First approach
Php open source ecommerce. First naive implementation, I like to use sets everytime I can. In range I used m+2 in to prevent set being empty and throwing an error when using min function.
Results:
- Task score - 88%
- Correctness - 100%
- Performance - 75%
I received TIMEOUT ERROR in medium performance tests (chaotic sequences length=10005 (with minus))
Second
Here I did better, first filtering for only positive numbers an returning 1 in case of an empty set. Then I use python built-in set symmetric difference.
Results:
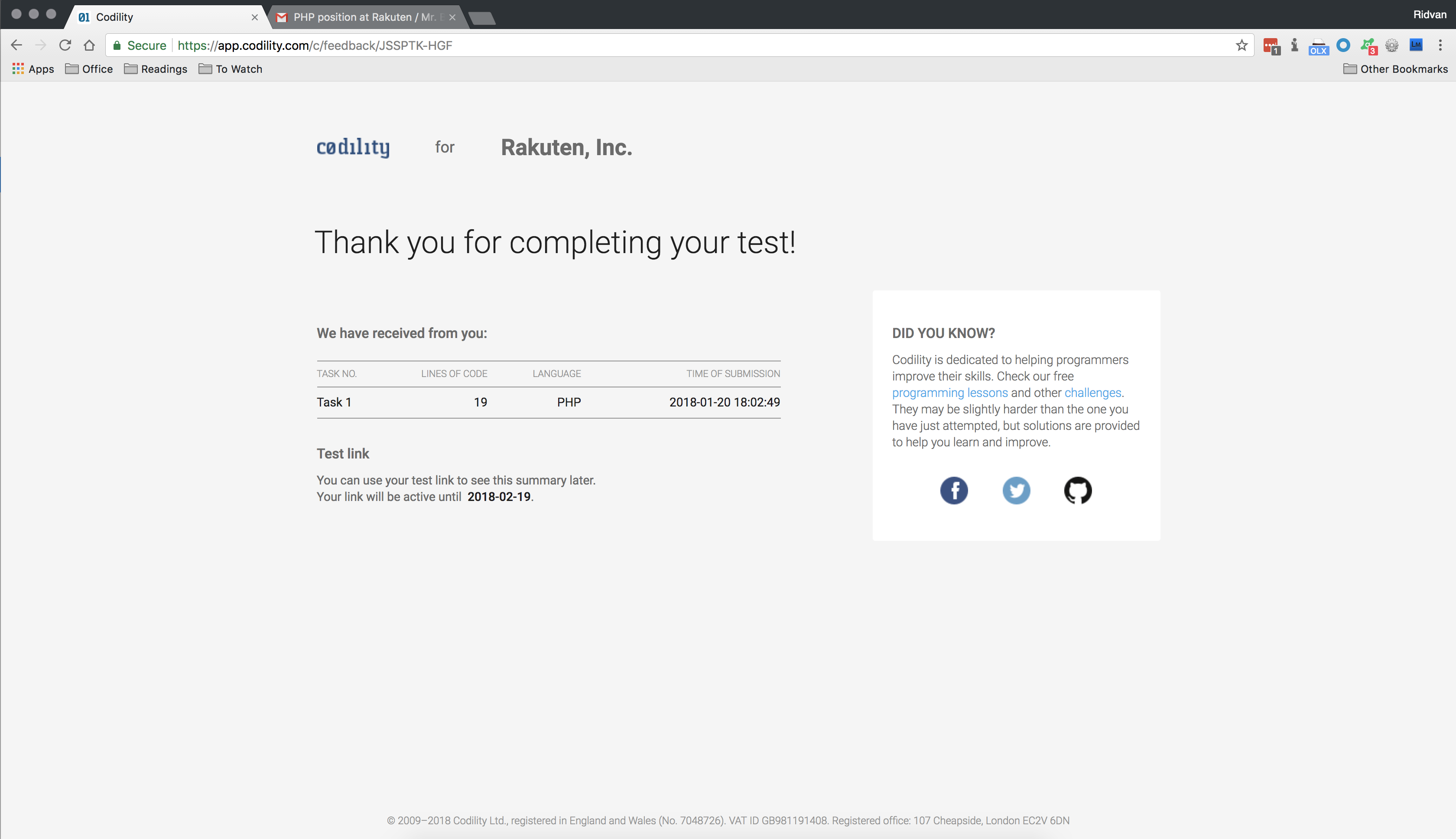
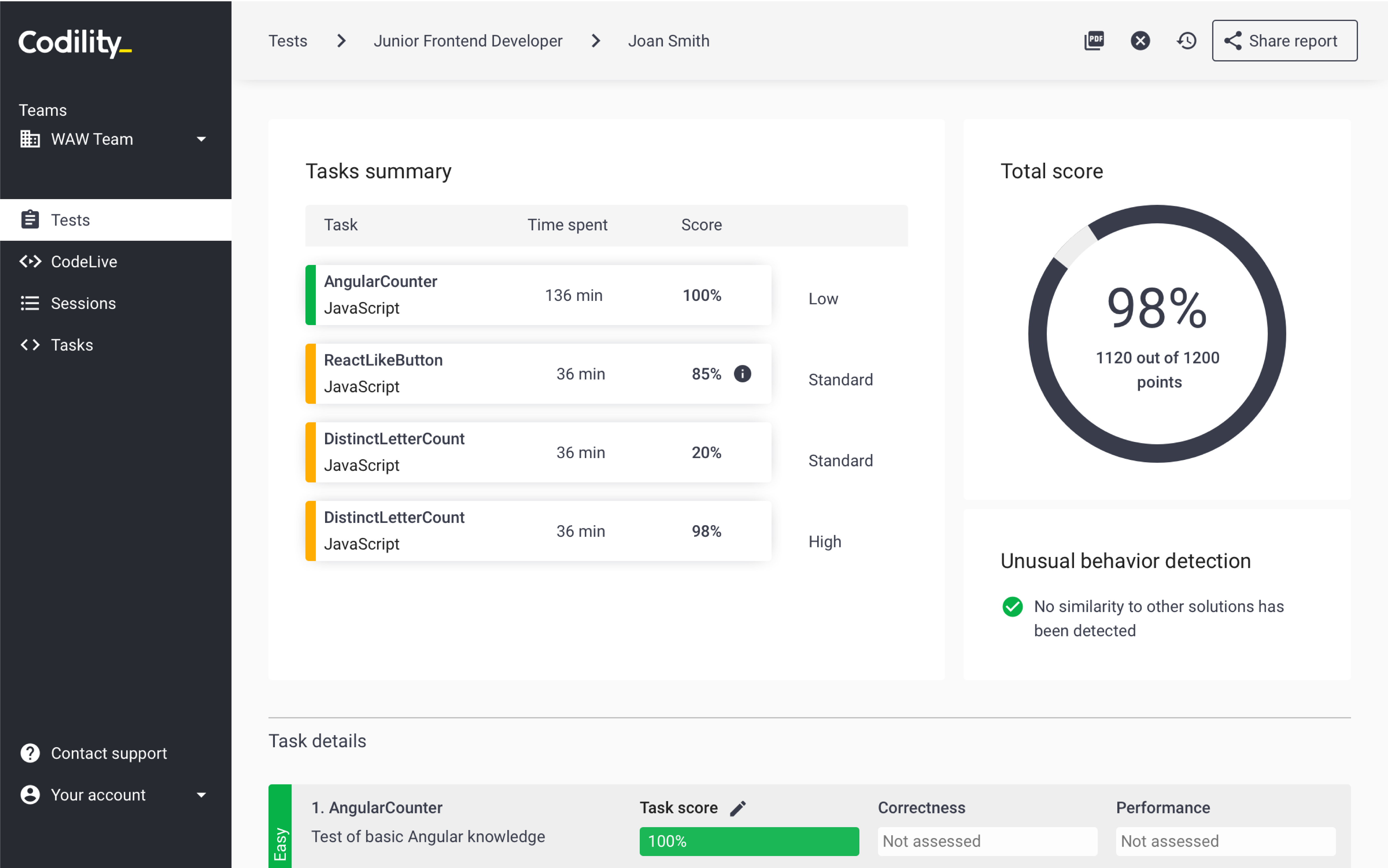
- Task score - 100%
- Correctness - 100%
- Performance - 100%
Detected time complexity: O(N) or O(N * log(N))
Third
My girlfriend approach results in time complexity worse than O(N), because of using sorted. Still, I find it interesting.
Results:
- Task score - 100%
- Correctness - 100%
- Performance - 100%
Detected time complexity: O(N) or O(N * log(N))
Fourth try: How to make O(N) ?
Looking for the solution, the best answer from stackoverflow was to use pigeonhole principle. I found a ready answer on nkbits blog with that approach, and the author claims that solution should be O(N) in time and space complexity. When analyzing tha code it looks he is right, but after running demo test in codility, I still got O(N) or O(N * log(N))… Why? Is this Python language fault or Codility server error?
Results:
- Task score - 100%
- Correctness - 100%
- Performance - 100%
Codility Test Tips Test
Detected time complexity: O(N) or O(N * log(N))
Several weeks ago I started passively looking for a job in the UK for the first time after not extending my contract. The process is absolutely fascinating and deserves a whole series of posts filled with things everyone has probably covered already. While some of the latest observations need to mature on the shelf before I share them, coming face to face with the evil of online testing of basic coding skills, Codility in particular.
In a nutshell, it is an online service where employers pick a set of coding challenges to present to candidates for a certain position and the candidate has a fixed amount of time to complete the challenge (once the timer starts it cannot be paused). The code is then tested by predefined tests and a summary of results is presented to the potential employer. They also get to see the timeline of changes the candidate made on their way to submitting the results. All of the tasks are tested for correctness (including all possible edge cases), most are also tested for time complexity and space complexity. Naturally, the analysis of results included a breakdown of how the candidate performed on each criteria.
Sounds like a very promising service, to a non-technical C-level executive perhaps. It should save a lot of effort interviewing candidates who are not even worth the time. It should also provide a nice evaluation of the candidates basic problem solving skills. The change timeline should illustrate the candidate's way of thinking. The home page of the service also states proudly that the testing matches the employer's job descriptions.
One of the top search results for 'Codility tests' is, ironically, a blog post titled 'Why I refuse to use Codility and so should You' (very short, recommend to read post and comments) which is a slightly more radical version of what I am trying to say. What I am writing is by no means a rant of a loser who failed their tests, I (surprisingly, at least to myself) pass. Still, hearing someone mention 'Codility' makes me cringe. So what could possibly be positive about these tests? Let us start with a summary of what I think is wrong with the approach.
1. The fact that it lets you filter out candidates uncapable of 'basic problem solving' (come to that later) is an illusion. People learn to cheat and lie at the age of 3 if not earlier. The value of 'being honest with yourself' comes much later if at all. I see no reason someone who wants the job too bad wouldn't just ask a very smart friend to do this on their behalf, visualising the (im)possible court proceedings on a case where a user has violated the terms and conditions of the service makes me giggle. Add that to the Googling and copy-pasting skills the candidate posesses and the probability of filtering out the bad developers is equal to meeting a dinosaur out in the street - maybe you will, maybe you won't.
2. Idenfitying basic problem solving skills is another thing the service is not helpful with. Let us look at the sample challenge and try to remember the last time we solved a problemformulated in such a way. Exactly. If you have that as a problem you just open a big fat reference book on Algorithms, pick the right one and translate the pseudo code into the right programming language. It is not a real world problem, the developer does not solve it but merely picks an algorithm and googling or browsing through a book is not really a skill. Not to mention the fact that the problems are stated in 1-3 screen statements more td;lr than this post.
3. My third problem with the tests is the environment. I would honestly rather write pseudocode on paper than write real code in Notelad and expect it to compile. And not just compile, produce the desired results without any unit tests. They do provide a proprietary testing facility which I sense is just a diversion to make you lose a lot of time figuring out how to use it. This tests very well if you are able to memorise all the APIs of the language you're using by heart and keep track of the loose brackets and braces, it also helps to test how fast you're able to copy and paste between your IDE and the browser.
4. Which takes us to the glorious functionality which allows to see how the candidate changed their code over time. Now let us assume they are, for some reason, not using their copy-pasting skills between the IDE and the browser and are indeed coding in the environment provided. Looking at someone code without hearing them talk about it, not to mention looking at their facial expressions, is pretty much like watching a video recording on mute: what was a 'Oooow!!! Heeeeelp!!!' may as well look like 'Go to hell!!!'
5. Filtering out people who are not worth the time is the strongest selling point and my biggest problem with the service. Having been on both sides of the interview I still believe software cannot be good at evaluating people. Software is good at solving repetetive tasks or at least tasks where there's a pattern and any amount of machine learning cannot compete with the experience of a single human being. A piece of software cannot tell you who is not worth your time, it cannot even give you enough input to make that decision. I have seen this before from the employer's perspective when a person with a resume which did not hit the right buzzwords turned out to be the perfect match during an interview. This week I was amazed at how two top executives in a successful company wasted 3 hours (on top of a 1h phone interview) on torturing me for a job which I had a sense was way out of my league. A very technical C-level and a technical IT manager from a company that has been successful for decades were prepared to invest their time into making sure I was not the right person although very promising an hour after I was prepared to shout 'I GIVE UP! Please let me go!' It does not prove anything statistically but it does make my opinions stronger.
So why should you NOT refuse to do a codility test?
Codility Test Questions
1. The first reason is very philosophical. Codility, no matter how evil, makes you challenge yourself. You don't get to see the right answer as a candidate (you do if you do a test assignment) and if you are one of those developers, it will leave you restless for a while, wondering what the 'right' way to solve it is. In a nutshell, it makes you openminded and self-conscious.
This comes at an expense: having no feedback and no access to the assignment itself to at least test it (unless you went through the trouble of copying it from sources while solving) leaves you wondering or even doubting yourself heavily. I was sure I was a failing looser and felt miserable when one of the companies failed to return any emails for 3 weeks only to find out I did pretty good in the end. On the bright side it made me think about the gaps I had in my knowledge and skills, buy some new books, do some research and some coding just for practice.
2. The second reason is very politicaland might help you a lot if you want to join the exciting sly and smily world of banking or corporate banking. Looking at how the potential employer evaluates your results or asking for feedback on your results during a face to face interview will tell you a lot about the people you will work with. Unless the results are processed by the HR based on the result numbers in which case you would quite likely not want to work for that company.
3. The most important benefit of these tests is the same as the benefit of doing any kind of tests. It is a type of yoga for your mind - you learn to bend your mind in all possible ridiculous ways which you will not probably need in real professional life but it makes you more ready for anything you might encounter. Very much like all types of IQ tests which I despised but learned to solve really quickly to stretch my mind.
To sum up, I will not refuse to do any tests and would not advise you to, however, if someone asks you to, you should try to extract as much information from these tests as the potential employer does.